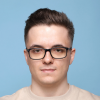
How Does Java Enable High-Performance?
Today, travel from Europe to Australia takes about 16 hours on a direct Qantas flight – a journey that 100 years ago would take over a month. Nowadays, speed is everything, and that principle applies to software development as well. In the presence of dynamic native and web applications, no user is going to wait for content to load. That poses a real challenge for software developers. So why, from tens of popular options, should you choose Java programming language when developing high-performance software? In today’s post, we are going to attempt to answer this question. We are going to focus on aspects of Java that make it perfect for low-latency and high-throughput systems. Keep reading!
Java Virtual Machine – the foundation of Java performance
At the core of Java performance lies Java Virtual Machine, or JVM for short.
The JVM has two main functions: to allow Java programs to run on any operating system or device (according to the well-known “write once, run anywhere” principle), and to optimize and actively manage program memory.
We can differentiate between two definitions of JVM, a causal and more technical one:
- Technical definition – The Java Virtual Machine is the runtime environment for a software program to execute its code.
- Casual definition – The Java Virtual Machine is how Java programs are run. We configure the settings and then rely on the JVM to manage program resources during execution.
There are a lot of elements that go into JVM, and each of them has a profound influence on the software application performance. We can distinguish three distinct components of JVM that has an effect on performance and which developers can tweak:
- Class Loader – When compiling a .java source file, it is converted into byte code in the form of a .class file. When you want to use this class file in your program, it has to be loaded into the main program memory by the class loader. This process involves 3 phases: Loading, Linking, and Initialization, all of which have a profound influence on the app’s performance.
- Runtime Memory/Data Area – Consisting of 5 core parts, it is responsible for providing memory to store the Bytecode, parameters, objects, return values, and local variables.
- Execution Engine – It is responsible for executing the code present in each class. However, before executing the program, the Bytecode itself needs to be converted into instructions that the JVM will understand. To achieve this, JVM can use an interpreter or a JIT compiler, which we will describe in more detail below.
JVM is a key tool for enabling true Java performance. An experienced developer can fine-tune Java Virtual Machine by adjusting its default parameters to better match application’s needs. This process can include adjusting the size of the heap, and choosing the right garbage collector.
As a general rule of thumb, when tuning a JVM you should first focus on the memory usage requirements, then on latency, and finally, on application throughput.
[Read also: How is Java Used in Software Development: The Ultimate Guide]
What makes Java a perfect language for high-performance systems
Ok, so we’ve established that JVM is a key tool for enabling Java performance. What about other aspects, characteristics, and functionalities that make Java a perfect programming language for building high-performance software applications?
Here are five key points:
1. Compiled and Interpreted
Programming languages can generally be either compiled (C, C++, Haskell, Erlang or Rust) or interpreted (PHP, Python Ruby, and JavaScript).
Java is, in that respect, a sort of jack-of-all-trades, as it combines the power of Compiled Languages with the remarkable flexibility of Interpreted Languages.
As we’ve already mentioned before when describing Java Virtual Machines, the Java compiler (javac) compiles the Java source code into the bytecode, which is then executable on all machines with JVM.
This diagram better visualizes this process:
2. Platform Independence
JVM gives Java its biggest advantage – platform independence.
Java Virtual Machine can be installed on virtually all available operating systems, from Windows, to Mac and Linux. Platform-Independence allows for compiling and executing code on any machine and ensuring the same results.
Bytecode is in this case the key to achieving full platform independence.
It is also worth explaining what Bytecode really is since we’ve already mentioned it a couple of times in this article.
Java Bytecode is simply a program that contains instructions for JVMs. It works similarly to an assembler, which is a representation of C++ code. In itself, it is a code in a binary format that consists of constants, references, and numeric codes which are readable and executable by the machine’s hardware.
Here is a diagram explaining how Java Bytecode allows for Platform Independence:
3. JIT – just-in-time compiler
And speaking of compiling, here’s where Java has another ace up its sleeve – the Just-In-Time compiler.
The way of converting Bytecode to native machine language for execution has a huge impact on its speed of it. JIT compiler interact with the Java Virtual Machine (JVM) and turns Java Bytecode sequences into native machine code.
The important thing is that the JIT compiler compiles code on-demand basis. That means that it compiles only a method that is being called. This greatly improves overall efficiency and saves time.
While using a JIT compiler, the computer hardware is able to execute the native code directly, as compared to having the JVM interpret the same sequence of Bytecode time after time. If compiled methods are executed quite frequently, this can lead to substantial performance gains.
What’s more, the JIT compiler is also able to perform a lot of simple optimizations while compiling to native machine language. Some of these optimizations include data analysis, translation from stack operations to register operations, reduction of memory accesses by register allocation, and elimination of common sub-expressions.
[Read also: Top Java Performance Problems and How to Solve Them]
4. Multithreading capabilities
Java is a technology capable of multithreading at a language level.
Multithreading allows programs to perform concurrent calculations on multiple computing cores and threads. What’s more, the multithreaded application can remain responsive to input, even while performing long-running tasks.
Multithreading is now more important than ever, especially when hardware companies are releasing ever more robust enterprise-grade CPUs like newly revealed Zen 4 Epyc processors, offering a mind-blowing 192 computing threads.
It is also crucial to differentiate between Multithreading and Multiprocessing. The first term refers to the ability to execute calculations on multiple CPU threads concurrently. The latter on the other hand refers to the ability of a system to run multiple processors concurrently, where each processor can operate multiple threads.
Generally, multithreading is preferred because the CPU threads use a shared memory area, which helps with memory conservation and allows for slightly faster content-switching.
5. Garbage collection
Last but not least, we have to cover the Java garbage collection.
In a nutshell, it is the process by which Java programs perform automatic memory management. When Java programs run on the JVM, objects are created on the heap but eventually, some of them will no longer be needed.
The garbage collector automatically detects these unused objects and deletes them, freeing up valuable memory resources in the process.
There are three phases of performing the garbage collection process in Java:
Checking for Eligibility
An object is eligible for Garbage Collection (GC) if it is unreachable.
We can distinguish four main ways of making a Java object eligible for garbage collection.
- Re-assigning the reference variable
Employee employeeOne = new Employee();
Employee employeeTwo = new Employee();
employeeOne = employeeTwo; // the first object referred by employeeOne is available for garbage collection
- Nullifying the reference variable
Employee employee = new Employee();
employee = null;
- Using an anonymous object
register(new Employee());
- Island of Isolation (group of objects that reference each other but are not referenced by any active object in the application).
Requesting JVM to run Garbage Collector
Requesting JVM to run Garbage Collection can be set up in two main ways:
- Using System.gc() method – System class contains static method gc() for requesting JVM to run Garbage Collector.
- Using Runtime.getRuntime().gc() method – Runtime class allows the application to interface with the JVM in which the application is running. By using its gc() method, we can request JVM to run GC.
Finalization
Just before deleting an object, Garbage Collector uses the finalize() method on the object to perform final cleanup activities. Once the finalize() method is completed, Garbage Collector deletes that object.
[Read also: Guide to Java Profilers: Which Performance Monitoring Tool Is the Best?]
How does Java enable high performance – summary
Java enabled high-performance software development as no other programming language does.
All developers should make sure that the Java program that they are working on is performing to its full capabilities, and properly leveraging all tools that this fantastic programming language has to offer.
Related Posts
We are Stratoflow, a custom software development company. We firmly believe that software craftsmanship, collaboration and effective communication is key in delivering complex software projects. This allows us to build advanced high-performance Java applications capable of processing vast amounts of data in a short time. We also provide our clients with an option to outsource and hire Java developers to extend their teams with experienced professionals. As a result, our Java software development services contribute to our clients’ business growth. We specialize in travel software, ecommerce software, and fintech software development. In addition, we are taking low-code to a new level with our Open-Source Low-Code Platform.