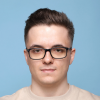
Java Best Practices That Every Java Developer Should Know in 2024
Java for the past years has been holding top spots among the most popular software development languages in the world. For good reason.
Java is a powerful, object-oriented, secure, and highly scalable programming language supported by a thriving community and a myriad of regular updates. It is no surprise that nearly three decades after its release, it is still an incredibly powerful software development tool. The question thus arises: how to master Java in order to use it to its full potential?
In today’s post, we are going to cover the best Java programming practices selected by our experienced Java developers. These tips are especially helpful to programmers with some commercial experience wanting to push their coding skills to the next level.
Why Java is so popular?
Java is currently the fifth most used programming language globally. That says a lot. As many as 64.96% of respondents in the recent study indicated Java programming language as the most used in their everyday work. This is the ninth year in a row that Java has achieved such a high position.
There are numerous reasons why Java is so popular and why it is used in so many commercial applications.
Here are the key aspects of Java that experienced developers value the most in 2024:
Scalability
Java offers many tools for building scalable, enterprise software. It supports modularity, static checking, and advanced tools for analysis, and debugging.
Garbage collection
Java offers an automatic memory management mechanism, known as garbage collection, which greatly improves the speed of applications.
Cross-platform capabilities
Java is cross-platform capable, which means that a compiled program can be easily run on different devices. Java compiler is the key to this cross-platform capabilities.
Multi-threading
Multi-threading allows concurrent Java applications to execute commands on multiple threads simultaneously, which greatly improves software performance and hardware utilization.
Active developer community
Thanks to Javaâs popularity, developers are provided with numerous sources of invaluable knowledge essential to their work.
Energy efficiency
Java is one of the fastest and most energy-efficient object-oriented programming languages.
Java’s role in the software development market – statistics
As of 2024, Java is still used by 30.55% of developers worldwide meaning that its still, even after 30 years, one of the most popular programming languages out there.
According to The PYPL Popularity of Programming Language Index, in 2024, Java was the second most popular language in the world, and its use has grown by 1.2% compared to last year. Oracle argues that Java is still hugely popular, used by over 69% of full-time developers worldwide with 51 billion active Java Virtual Machines (JVMs) deployed globally.
Javaâs contribution was over 31% in 2008, and still, it holds 15.81% in 2024. You might say that’s quite a noticeable decline but in reality, Java is still a foundation of many enterprise software solutions around the world.
Why?
It provides a great mix of performance, ease of use, and scalability – all things that modern businesses need to thrive.
How can I improve my coding skills in Java?
So you have some commercial experience in Java software development but strive to improve your coding skills even further?
Great!
Here are a couple of basic rules and advice to follow:
Get your basis cleared
Before diving into the more advanced stuff, you have to make sure that you are familiar with all the basic concepts and know all the necessary tools like IDE’s and frameworks. Upon that foundation, you can then expand your knowledge.
Remember that rather than struggling with something that you donât get, seek help. There are a myriad of invaluable sources of knowledge like YouTube tutorials, books, Stack Overflow forums, and the most important of them all, help from your colleagues at work.
Read Documentation of new updates and stay up to date
Every good Java developer should be familiar with what’s happening in the Java ecosystem.
Keep yourself updated with the latest Java news and updates by joining good forums, following industry experts on LinkedIn, and subscribing to newsletters.
Always strive for knowledge
You won’t succeed as a Java software developer if you stay in one place.
There are many different technologies, integration tools, and frameworks in the Java ecosystem. If you want to become an expert in this field, your tech stack should be as comprehensive and versatile as possible.
Practice coding
Practice makes perfect!
There is no better way of improving your coding skills than working on development projects.
Try to implement what you are learning. Practice programming online or offline, thatâll boost your confidence. Be regular, make targets and try to complete them within a specific time period.
What are the main Java development principles and coding standards?
For Java programmers to write code that is clean, readable, and bug-free, they can follow a couple of well-established Java programming principles that are the backbone of high-quality software. Here are five simple Java coding standard for Java software developers:
KISS, DRY, and YAGNI
These rather cryptic acronyms stand for three valuable rules of thumb in software development and go as follows:
- Keep It Simple, Stupid – Implementing complicated and ambiguous software architecture just for the sake of being innovative is a common mistake among developers. In every situation, Java programmers should look for the simplest solution and apply it in practice.
- Donât Repeat Yourself – Developers should try to avoid duplicating parts of code by putting it into a single system or a method.
- You Ainât Going to Need It – The volume of code and functionalities should be dictated solely on the basis of strategic plans, rather than on-the-spot decisions.
Prioritize clean code over clever code
Using fancy functions and advanced solutions should never come at a cost of code readability.
Avoid premature optimization
True bottlenecks will become apparent at the end of the development cycle, and that’s when optimization efforts should commence.
Single responsibility
Every module or class in software should only provide one bit of specific functionality.
Use Fail fast, fail hard approach
The fail-fast approach stands for stopping development as soon as any unexpected error occurs, which allows for deploying high-quality code faster.
What are Java best practices for developers? 11 tips from our experts
Now we can move on to the things that you can do to really help improve your practical coding skills.
Here are 12 of the best Java coding practices and Java coding standards for 2024 selected by experienced Stratoflow developers:
1. Use clear and intuitive Naming Conventions
First things first. Before diving deep into the project, you should start by setting a proper naming conventions for every class, interface, method, and variable.
Keep in mind that more advanced software development projects can involve multiple teams of developers.
It is thus crucial to agree upon and follow one naming convention in order to maintain uniformity and avoid confusion.
Here are a couple of tips for setting up easily understandable and clear naming schemes:
- Classes – Names should only include nouns and begin with uppercase letters,
- Packages – Names should be entirely written in lowercase,
- Interfaces – Names should follow Camel Case,
- Variables – Names can follow the mixed case convention,
- Methods – Names should consist of verbs with every word capitalized.
You might think that these recommendations are rather insignificant. In reality, though, after multiple merges and adding new functionalities, the convenience will be clearly evident.
After all, a proper naming scheme is even more important than excellent code comments. This is because Java programmers often neglect that part of their work and forget to update the comment when changing the code, leading to further confusion.
2. Remember about commenting and writing self-documenting code
Since we’ve already mentioned it, let’s now move on to one of the most often neglected parts of the software development process – commenting.
How does anyone reading a codebase understand it? First, they need to know what the code is âsupposed to doâ. That’s where comments come in.
Commenting is a practice of placing human-readable descriptions inside the code explaining what a particular part of the program is doing. As your code will be read by other team members with varying knowledge of Java, comments should give them a clear overview of chosen approaches, and provide additional information that isn’t easily understandable just by looking at the code. Good comments can greatly simplify Java code maintenance, as well as help diagnose and fix bugs much quicker.
Some developers go even a step further and make an argument for self-documenting code.
This is a kind of code written in a way that doesnât need separate documentation, as it is virtually self-explanatory to the reader.
Here is an example of an ordinary comment in Java code:
// check if employee has right to all benefits
if ((employee.flags & HOURLY_FLAG) && (employee.age > 65))
Self-documenting code greatly improves overall readability and makes your code cleaner. In this sample a developer can clearly see what is being done:
if (emplyee.isEligibleForFullBenefits())
Nevertheless, self-documenting code works best in tandem with additional comments that better explain why it is being done.
One of the Stratoflow Java developers pointed out the key advantages of writing clear, self-documenting code:
My colleagues have a much easier time with code reviews. And also if I have to come back to a project in the future and I don’t remember anything about it, such code is invaluable, as it helps to grasp the topic faster, and it doesn’t make me angry at myself for my carelessness.
3. Make sure commits are well described
Code and variables are not the only elements of the software development process which have to be properly described. The same rule applies to the commits as well.
Besides the main content of your code, a commit also stores various additional information like the author of the commit, timestamp, and a message. The latter should not be disregarded, as it provides other team members with valuable information about what was done in the repository over time.
An example of a git commit message is:
git commit -m “Improve performance with lazy load implementation for images”
There are a couple of best practices concerning writing a good commit message that every Java Developer should be familiar with:
- Capitalize the first letter of the commit message, i.e. “Fix bug with global search input deleting user text”
- Keep it brief and straight to the point. It is generally advised to keep a commit message under 50 characters.
- In your commit message, focus on what has changed, rather than why you changed it.
4. No Empty Catch Blocks
Let’s finally move on from the comments and descriptions, and let’s cover some practical coding tips, practices and Java coding standards, starting with Catch Blocks.
Java Try catch block is used for handling exceptions. Written code that can throw an exception is placed inside the try block and if a certain exception is thrown, it is handled by the corresponding catch block. So if anything unplanned happens in the try block, for instance, divide by zero, file not found, etc. it will generate an exception that is dealt with by the catch block.
In theory, we can have an empty catch block. In reality, though, this is a bad practice and should be avoided. An empty catch block won’t give you any information about what went wrong within our code and prolong the bug-fixing process.
Consider the following code snippet:
public int aggregateIntegerStringss(String[] integers) {
int sum = 0;
try {
for (String integer : integers) {
sum += Integer.parseInt(integer);
}
} catch (NumberFormatException ex) {
}
return sum;
}
In this example, every catch block is empty and when we run this method with the following parameter [â123â, â1abcâ]
The method will fail silently without giving us any feedback and return 123.
Depending on the situation, the code for handling exceptions may be different. Nevertheless, it is worth remembering to never ignore an exception by creating an empty catch block.
5. Proper handling of Null Pointer Exceptions
Let’s now cover a really common exception in Java – NPE or Null Pointer Exceptions.
A Null Pointer Exception is thrown when a program attempts to use a string object reference that has a null value. Such a situation can happen when:
- Modifying or accessing a null objectâs field,
- Invoking a method from a null object.
- Modifying or accessing the slots of a null object.
- Throwing null, as if it were a Throwable value.
- Synchronizing over a null object.
Coming across Null pointer exceptions is inevitable when working as a Java software developer, but it is worth following some basic guidelines in order to handle them properly.
First and foremost, check possible Nulls values and variables prior to code execution so that they can be eliminated. You should also modify your code in order to better handle exceptions.
Consider this short code snippet:
int noOfworkers = company.listWorkers().count;
Theoretically it shouldn’t happen, but if either âCompanyâ or method âlistWorkers()â is a Null, the code will throw an NPE.
A better version of this could look like this:
private int getListOfWorkers(File[] files) {
if (files == null)
throw new NullPointerException("File list cannot be null");
6. Use Java libraries ergonomically
One of the main reasons why Java still holds the spot as one of the most popular programming languages worldwide is its support for the vast variety of code libraries. Known as Java Class Libraries, these sets of pre-written code are essential tools for software architects. Using them, Java programmers can assemble parts of code faster without the need for writing every single function manually.
It is worth noting, though, that using too many libraries is a bad practice in software programming and can prove detrimental in the long run.
It’s because each library requires dedicated system resources to be loaded. Apart from using substantial amounts of system memory, they can also hinder the applicationâs performance. There’s also an issue of libraries’ quality. Some of them include buggy code which can do more harm than help.
It is a good practice for Java programmers to use only these libraries from trusted sources that fulfill multiple purposes in order to save valuable memory resources.
7. Class Members must be accessed privately
According to the author of Effective Java Joshua Bloch, the accessibility of class members should be minimized. Java developers should thus use a private modifier to protect the fields in order to enforce information type in the software design.
Let’s consider these public fields:
public class Employee {
public String name;
public int age;
}
Due to the poor software design, field values can be changed inappropriately:
Employee employee = new Employee();
Employee.name = "";
Employee.age = 28;
Taking into consideration the type of data, these values don’t make much sense. A good practice is to hide these fields and allow the code to change them using setter methods or mutators. Here’s an example of a better approach:
public class Student {
private String name;
private int age;
public void setName(String name) {
if (name == null || name.equals("")) {
throw new IllegalArgumentException("Name is invalid");
}
this.name = name;
}
public void setAge(int age) {
if (age < 1 || age > 100) {
throw new IllegalArgumentException("Age is invalid");
}
this.age = age;
}
}
8. Avoid redundant initializations
Even though this is a pretty common practice among Java developers, we advise against initializing member variables with values: like false, o, or null.
These values are already the default initialization values of member variables in Java. It is a good Java best practice to be aware of the default initialization values and avoid initializing other variables.
9. Avoid memory leaks
There are two things that can significantly influence software performance – memory leaks and object creation.
Generally, Java developers do not have much control over memory management, since Java uses an automatic system for that. While it’s cool not to have to worry about freeing and allocating memory manually, it doesnât mean that Java developers should not pay any attention to how memory is used in the application.
It is a good programming practice to always release database connections after querying, using Finally block as often as possible and releasing instances stored in Static Tables. All of these tips are effective ways of preventing memory leakages.
There are also a couple of tools to help you detect memory leaks in your code.
- Memory tab in IntelliJ IDEA – IntelliJ is one of the most popular IDE in Java development and there’s a high chance that you are using it in your everyday work. It also has an in-built feature that allows you to view details of all objects, which is really helpful in detecting memory leaks and their causes.
- NetBeans Profiler – It can be used to analyze memory usage in Java FX, Java SE, EJB, mobile and web applications.
- Memory Analyzer (MAT) in Eclipse – It allows Java developers to detect possible memory leakages and easily analyze the heap dump, even if it contains millions of objects.
10. Float or double?
Some insufficiently experienced Java programmers have the tendency to use double and float in inappropriate applications. They usually choose only one type, regardless of the project guidelines.
If you need precise calculations, you should use double instead of float. Most CPU’s take a similar amount of time to process operations either on a float or a double, but double value offers much higher precision than float.
When precision is not as important, a good practice is to use float values instead of double because it takes up half the memory space.
A good Java developer should always remember that using the right data type significantly improves the performance of your code.
11. Make sure that the code is well-covered with tests
Last, but certainly not least, we have to cover software tests.
Software testing is a method of determining whether the actual software product meets the expected requirements and ensuring that the software product is free of defects. It is often regarded as one of the most tiresome chores in software development.
But don’t neglect it!
Code that hasn’t been properly covered by tests is notoriously hard to maintain. A developer may never be sure what part of the project is about to blow up if there is a slight change made to the code.
It is best to strive for the best test coverage possible, but you don’t have to go for 80-90% coverage right away. First, focus on the most important areas of the project, and the ones that are the most vulnerable to failures and bugs.
Java coding best practices – summary
Java is an extremely versatile and powerful programming language that has found its use in a multitude of industries, from fintech and e-commerce to gaming and entertainment.
In order to use its vast capabilities to their full potential, programmers need to learn to not just code but code smartly. It is crucial to create high-performance software and write code that is clearly readable to other team members, better composed, and free of bugs.
If you want to join our team of experienced Java developers and do cool stuff with cool people, join us.
Related Posts
We are Stratoflow, a custom software development company. We firmly believe that software craftsmanship, collaboration and effective communication is key in delivering complex software projects. This allows us to build advanced high-performance Java applications capable of processing vast amounts of data in a short time. We also provide our clients with an option to outsource and hire Java developers to extend their teams with experienced professionals. As a result, our Java software development services contribute to our clients’ business growth. We specialize in travel software, ecommerce software, and fintech software development. In addition, we are taking low-code to a new level with our Open-Source Low-Code Platform.