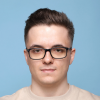
Java Clean Code: How to Write It? Best Practices From Experts
Software development is a complex craft.
There are always numerous ways of writing certain parts of code or designing new functionalities. Ultimately, they might do the same thing, but some might be orders of magnitude more efficient and easy to understand than others.
It’s up to developers to figure out what approach will work best and how to write high-quality clean code.
In this article, we are going to focus on writing clean code in Java. Why is it so important to write easily readable code? What are the best practices for developing code in Java? Keep reading to find out!
Why do you need clean code in Java?
Let’s start with defining what clean code really is.
Clean code might mean different things to every programmer, so it is really quite difficult to pick a single definition. Nevertheless, we can say that clean code is most certainly straightforward, and easy to read and comprehend.
It is also well organized and follows all the most important conventions and good practices of the Java programming language. But probably most importantly, clean code is free of errors and bugs.
The general idea behind clean code is pretty simple. We as programmers don’t really write code for the machines.
What do we mean by that?
Well, a computer can easily execute a convoluted codebase as long as it is free of bugs. Humans on the other hand are not that one-dimensional. We have to put effort into understanding the logic behind the code that someone else has written.
More often than not, getting a grip on the existing codebase is a substantial chunk of the developer’s work. Some even say that the profession of a software developer is more about reading than developing, because 80% of our time is spent doing just that – reviewing and understanding someone else’s code.
And there’s no easy workaround. Even with minimal team turnover, there’s no way for the developers to be familiar with every part of the codebase in larger, enterprise-grade programming projects.
And that’s why clean and maintainable code is so important – to make arguably the most time-consuming task of a developer – reading a project’s codebase – a bit more bearable.
How to write clean code in Java?
To write clean code, you need to have a rather good knowledge of the Java language and its syntax.
But don’t worry!
You don’t have to be a senior developer with tens of years of experience under your belt to write clean code.
All you have to do it’s to always keep in mind these three basic principles of clean code and try to apply them in practice whenever possible:
- Simplicity – You should strive to make your code as simple as possible. Unnecessary complexity will only make the code hard to understand and prone to errors.
- Maintainability – The code you write should be easy for other developers to work on and maintain it. Basically, the whole application should not break down if you change some of its components.
- Testability – Unit tests are an integral part of writing clean Java code. It is good practice for developers to cover the code they write with automated tests right from the beginning.
How to improve Java code readability?
The first associations with the term “coding” that come to our minds are probably technical and related to ciphers and machines.
The reality of software application development positions should be rather different. Java programmers should not write code that is just understandable to the computer. Instead, they should write code that is just as easily readable for their work colleagues as well.
Just like a Polish IT expert and coach, Sławomir Sobótka has said in one of his Ted Talks,
Don’t code, write prose.
To check whether your code is sufficiently human-readable, you can do something called a speech synthesizer test. Just paste some snippets of your code into a speech synthesizer (or just read it out loud) and check whether there will be problems with smooth pronunciation. If so, look for improvements. Maybe some of your methods are too long? Maybe the names of objects and classes are too complicated? The possibilities are virtually endless.
What are the limits of clean code?
Alright, so we’ve already established that clean code is easy to read and free of bugs. But can you write code so clean and simple that it becomes self-explanatory?
Well, not really.
You might be tempted to get rid of code comments when you focus so much on making your code as clean as possible. After all, if the names of the classes and variables are clear and easy to understand, why would you need them?
This is a big mistake.
Comments and documentation are used to answer the question “Why?”
Why is this implemented like that? Because of the client’s requirements listed in the attached doc file.
Why does this return a null value instead of throwing an exception? Because the library, it is a callback for, expects that and doesn’t trap exceptions.
Why do we need these three versions of the initializer? Because A needs the first one, B needs the second one, and C is for someone else’s code.
And so on. A code-only implementation would explain none of this. A key takeaway from this is that clean code is not a substitute for proper documentation. Its main objective should always be to make the codebase more easily readable for your fellow teammates.
Java clean code best practices – 8 tips from our experts
Now we know what the clean code really is and what are its benefits. Let’s move on to some tips on how to improve the Java code quality from our most experienced developers.
1. Maintain a sound project structure
First and foremost, a key to writing high-quality clean code is to maintain a sound project structure to reduce search time among files. There’s no top-down rule when it comes to setting up the project structure, but there are certainly some good practices to follow.
Even though you choose your own pattern, several Java build tools such as Maven suggest a specific structure. Maven proposes these four folders that developers should include in their projects.
- src/main/java – It contains the source file structure of the project.
- src/main/resources – It contains the resource files of the project.
- src/test/java – It contains the test source files of the project.
- src/test/resources – It contains the test resource files of the project.
2. Use simple Naming conventions
Another key principle is to use clear and describable names for variables, techniques, and classes. It makes it much easier for other developers to understand what your code does and make it flow like sentences in a book.
It also makes it easier to find bugs. In addition, using clear and descriptive names makes it easier to change your code later.
Developers should seek rather short names that are good at encapsulating what specific logic in code is responsible for. If you have code that uses object names with 3, 4, or even 5 words in them, it becomes increasingly hard to read. It is thus worth striving for this golden means in the form of short names that are good for describing what each part of the code does.
After all, a feature that made Java stand out from other programming languages like C, was the fact that it had human-readable object names. Let’s not waste that by needlessly complicating them.
A good tip for non-native English-speaking developers is to look through the dictionary or thesaurus when searching for names for various methods and classes.
3. Code reviews are your friend
Code reviews are considered one of the most time-consuming and tiresome chores of software developers.
But when it comes to writing clean code, it shouldn’t be perceived that way. Actually, code reviews should be your best friend when striving to write code that is easily readable to others.
Generally, code reviews are important parts of the software development process, as programmers get a second opinion on the solution and implementation before it’s merged into a main branch of the project. The reviewing sofware developer is often the second step in identifying bugs, discovered edge cases, bugs, and other issues.
But from the clean code standpoint, code reviews offer one more, incredibly helpful advantage.
They act as manual readability tests. Other Java developers read through your codebase and check whether it is clearly understandable to people unfamiliar with its logic.
So don’t get mad if a colleague that gives you their review, adds remarks to small things like spaces between the lines of code. You might think this is just nitpicking but in reality, little things like that greatly improve the readability of the code and should always be taken into consideration during code reviews.
4. KISS & DRY
It’s hard to make a list of good practices in Java coding without mentioning KISS and DRY.
The first one, KISS, means keep it simple, stupid. It basically tells developers to avoid making the code unnecessarily complicated. Functions should be small and rarely exceed 20 lines of code. What’s more, functions should also do only one thing stated in their name. Thanks to this practice, they are more or less guaranteed to be short and easily understandable to the human reader.
The second rule is DRY, or don’t repeat yourself. Code repetition is usually the root cause of a huge number of bugs and problems in software development. Duplicate code means that when making changes in the code’s logic, developers need to make changes in multiple different places – a true recipe for disaster. Developers should use their IDE’s refactoring features and extract methods whenever they see a repetition.
[Read also: How is Java Used in Software Development]
5. Maintain a good visual representation of your code
Would you enjoy reading a book in which each paragraph has different sizes of indentations, margins, and spacing? I’m sure that such a reading experience would without a doubt be unbearably annoying.
So why would we force it upon our colleagues who are doing a code review for us?
Things like dividing the lines of code, putting closing brackets the same way through the entire code, making proper indentation, or leaving an empty line between the methods might seem insignificant, but they can do wonders for the overall code readability.
And as we’ve already mentioned in the third point, don’t hesitate to point out little things like that in your code reviews. After all, developing good programming habits will benefit everyone in the long run.
6. Remember about unit tests
In order to do simple code refactoring, we have to have unit tests set up properly beforehand.
Why?
Well, it’s simple. If you tinker with your code to improve quality and readability, there’s always a risk of messing something up along the way. So the rule is simple – to have clean code, you must be able to refactor. And to refactor, you must have unit tests.
Besides that, unit tests, in a way, force developers to divide their code into conceptual parts. Thanks to that, it is much easier to understand what the code is supposed to do.
7. Write good comments and documentation
As we’ve mentioned at the beginning, some developers might be under the impression that clean code will make ordinary documentation obsolete. After all, clean code should be easily readable and understandable to all.
But no matter how clean you write your code, nothing will replace proper documentation comments.
But by documentation we mean proper documentation. Writing a comment to a field describing what’s literally in its name won’t cut it. Good documentation should go more into detail about the business logic of the project, explain some terms and principles that are not easily deducible from the codebase itself.
Based on our experience, the best way to maintain documentation is to have it in Javadoc comments, as they are easily accessible with the Ctrl+q shortcut. Additional information on what certain business logic does always improves code quality in the long run.
8. Clean code is not only for production code
Good programmers should write clean code not only meant for production, but for testing environments as well.
Developers often encounter projects where production code is of high-quality and written according to the principles of clean code. Test code on the other hand was just a pure dumpster fire.
So what to do to make your test environment clean as well?
Well, just as developers usually avoid writing methods longer than 20 lines, we should also do the same for test code. You should try not to make your test methods longer than approximately half of the space on the screen. It allows other programmers to better comprehend what is going on in more complicated business logic. Additionally, all other principles and good practices of programming should be applied there.
Tools for writing clean code
Automatic code formatting tools
For clean code, proper formatting is absolutely essential.
Luckily, popular code editors for Java offer some automatic tools and solutions. Thanks to them, programmers can save a lot of time on things like fixing proper spacing or closing brackets.
Static Analysis Tools
For Java, there are many static code analysis tools such as SonarQube, Checkstyle, and SpotBugs, offering a set of rules that can be applied to your projects to improve code formatting.
[Read also: What is a Tech Stack and How to Choose One?]
Clean code – further reading
There’s no way that we could encapsulate the whole topic of writing clean code in a 2000 word article.
If you want to learn more cool high-quality programming practices, we highly recommend checking out “Clean Code: A Handbook of Agile Software Craftsmanship” written by Robert C. Martin. It’s a great source of rules and guidelines on design, understandability, naming conventions, source code structure and so much more.
It’s definitely a must-read for any software developer who is looking to push their craft to a new level.
Closing thoughts
Good programmers write code that is easily understandable not only to the machine but to their teammates as well.
Of course, writing clean code is not a skill that you can acquire overnight. It is a product of months, or even years of practice, as well as learning basic principles and guidelines of programming craftsmanship.
Regardless, writing clean code is without doubt hugely beneficial to everyone involved: to the business, to other team members, and even to the developers themselves.
Related Posts
We are Stratoflow, a custom software development company. We firmly believe that software craftsmanship, collaboration and effective communication is key in delivering complex software projects. This allows us to build advanced high-performance Java applications capable of processing vast amounts of data in a short time. We also provide our clients with an option to outsource and hire Java developers to extend their teams with experienced professionals. As a result, our Java software development services contribute to our clients’ business growth. We specialize in travel software, ecommerce software, and fintech software development. In addition, we are taking low-code to a new level with our Open-Source Low-Code Platform.